전체 선택 기능을 구현하기 위해 부모 컴포넌트에서 자식 컴포넌트의 함수를 호출할 일이 생겼다.
전체 선택은 부모 컴포넌트에 있고, 각각의 플레이 버튼은 자식 컴포넌트 들에 있었다.
//부모 컴포넌트
const JamDetail = ({ match }) => {
const child = useRef([]);
const onCheckAll = (isChecked) => {
if (isChecked) {
const indexArray = data.map((music, index) => index);
setvalue(indexArray);
} else {
setvalue([]);
}
}
const allplay = () => {
value.forEach((index) => {
child.current[index].Playing();
});
}
const selectPlay = (e) =>{
if (e.target.checked) {
setvalue([...value, index]);
} else {
setvalue(value.filter((v) => v !== index));
}
}
return(
<>
<div>
<input type="checkbox" checked={value.length === data.length} onChange={(e) => onCheckAll(e.target.checked)} />
<span>전체 선택</span>
</div>
{data.map((musicInfo, index) => {
return (
<input type="checkbox" checked={value.includes(index)} onChange={selectPlay}/>
<Waveform
data={}
key={}
src={}
ref={(elem) => (child.current[index] = elem)}
/>
)}
}
</>
)
}
자식 컴포넌트
import React, { forwardRef, useImperativeHandle} from "react";
const Waveform = forwardRef((props, ref) => {
useImperativeHandle(ref, () => ({
Playing
}))
function Playing() {
setPlay(!playing);
wavesurfer.current.playPause();
}
}
1. 부모 컴포넌트에서 child useRef를 선언해 줍니다.
2.useImperativeHandle을 통해 사용할 함수를 정의해줍니다.
3. 부모 컴포넌트에서 사용할 때 child.current. []. Playing() 이런 방식으로 함수를 호출할 수 있습니다.
위의 예제코드를 통해 선택한 음원만 재생할 수 있게 구현을 해 보았습니다.
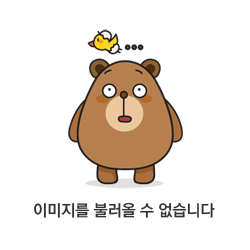